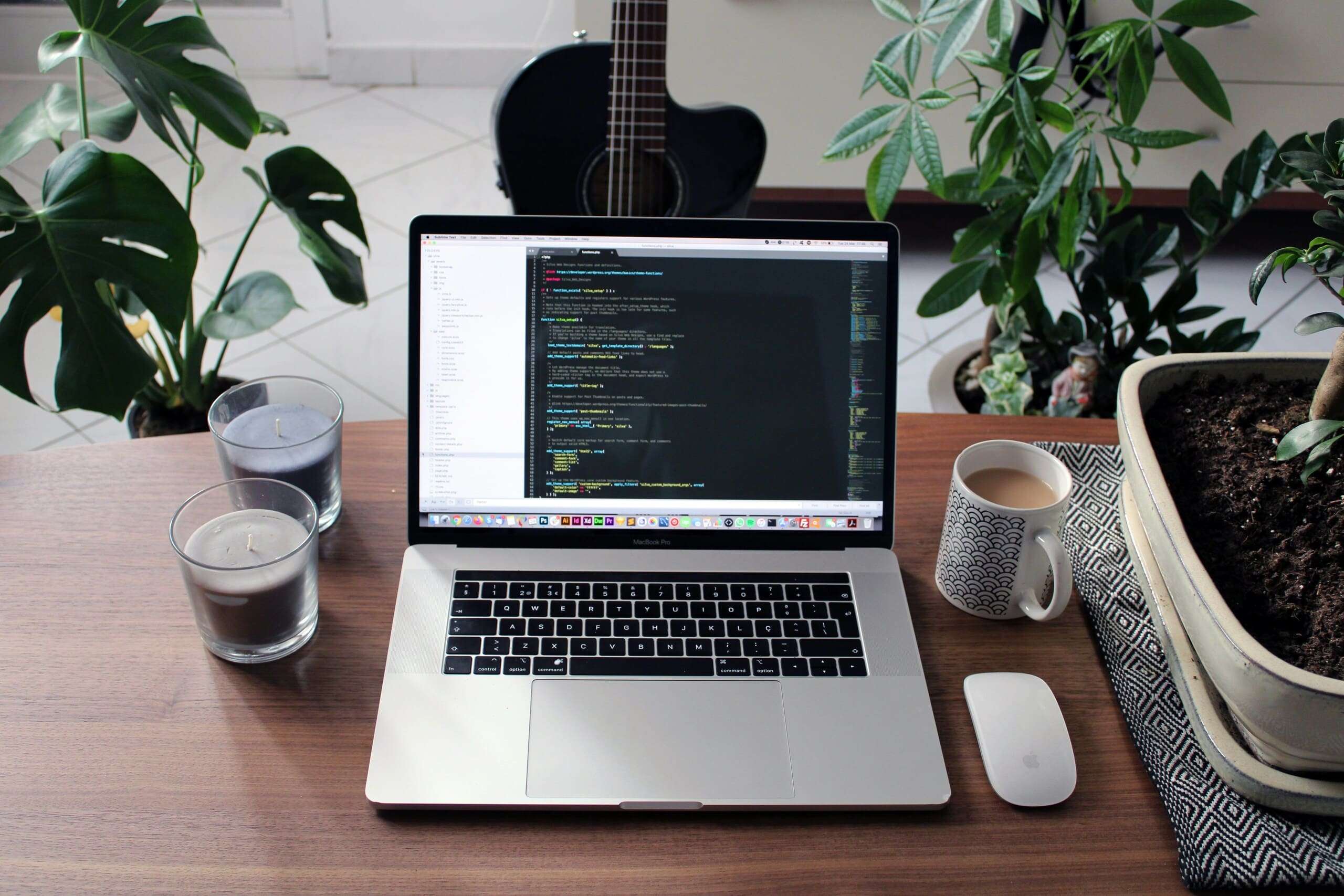
When building modern web applications with React, one of the major challenges developers often encounter is state management in React. As your application scales, managing how data flows and changes across components becomes increasingly complex. Fortunately, Redux in React provides a structured and efficient way to manage this complexity.
In this beginner-friendly guide, we’ll explore the basics of Redux, how it simplifies state management in React, and how you can integrate it into your own applications.
What is Redux in React?
Redux in React is a popular state management library that helps developers handle application state in a more predictable and centralized way. It enforces a unidirectional data flow, meaning the state only moves in one direction—from the store to the UI—making your app easier to debug and maintain.
At the heart of Redux are three core principles that improve state management in React:
- Single Source of Truth: The entire state of your app is stored in a single, centralized object called the store.
- State is Read-Only: The only way to change the state is by dispatching actions—plain JavaScript objects describing the change.
- Changes via Pure Functions: State updates are handled by reducers, which are pure functions that take the current state and an action, and return a new state.
These principles work together to create a clear and consistent structure for state management in React applications.
How to Set Up Redux in React:
To get started, install the necessary libraries:
Bash
npm install redux react-redux
1. The Store:
Once installed, you can create the Redux store, which holds your entire application state.
javascript
const { createStore } = require(‘redux’);
const store = createStore(rootReducer);
The rootReducer is a combination of all reducers in your app, each managing a portion of the state.
2. Actions: Describing State Changes
In Redux, actions are plain objects that describe what needs to change. Every action must have a type property and may include additional data via a payload.
javascript
const incrementAction = {
type: ‘INCREMENT’,
};
Actions are dispatched to signal a change in state, which is then handled by reducers.
3. Reducers: Handling State Changes
Reducers are pure functions that determine how the state should change based on the dispatched action.
javascript
const initialState = { count: 0 };
function counterReducer(state = initialState, action) {
switch (action.type) {
case ‘INCREMENT’:
return { …state, count: state.count + 1 };
case ‘DECREMENT’:
return { …state, count: state.count – 1 };
default:
return state;
}
}
Since reducers are pure, they always return the same output for the same input. This predictability is key to effective state management in React.
4. Dispatching Actions:
To update the Redux state, use the dispatch() method with an action:
javascript
store.dispatch({ type: ‘INCREMENT’ });
Every time you dispatch an action, the store runs the reducer function, which returns a new state. This updated state is then reflected throughout your React app.
Connecting Redux to React Components:
To integrate Redux in React, use the react-redux library. It provides two essential features: Provider and connect.
1. Provider
The Provider component wraps your application and makes the Redux store accessible to all nested components.
javascript
import { Provider } from ‘react-redux’;
function App() {
return (
<Provider store={store}>
<MyComponent />
</Provider>
);
}
2. Connect
The connect() function links your React components to the Redux store. It maps state and dispatch functions to component props.
javascript
import { connect } from ‘react-redux’;
import React from ‘react’;
const MyComponent = ({ count, increment }) => (
<div>
<p>{count}</p>
<button onClick={increment}>Increment</button>
</div>
);
const mapStateToProps = (state) => ({
count: state.count,
});
const mapDispatchToProps = (dispatch) => ({
increment: () => dispatch({ type: ‘INCREMENT’ }),
});
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
This pattern helps React components stay clean and focused on rendering, while Redux handles all the heavy lifting behind state management in React.
Conclusion: Why Use Redux in React?
To summarize, Redux in React provides a powerful solution for predictable, centralized, and scalable state management in React applications. While it may not be necessary for small projects, it becomes incredibly valuable in larger apps where multiple components rely on shared or complex state.
By following its core principles—single source of truth, read-only state, and pure functions—Redux brings clarity and control to your application’s data flow. Once you get the hang of it, you’ll see how Redux in React becomes an indispensable part of your development toolkit.
Talk to our solutions expert today.
Our digital world changes every day, every minute, and every second - stay updated.